Related Topics
JavaScript Objects, Events and Popup Boxes
Lesson 4 – JavaScript Objects, Events and Popup Boxes
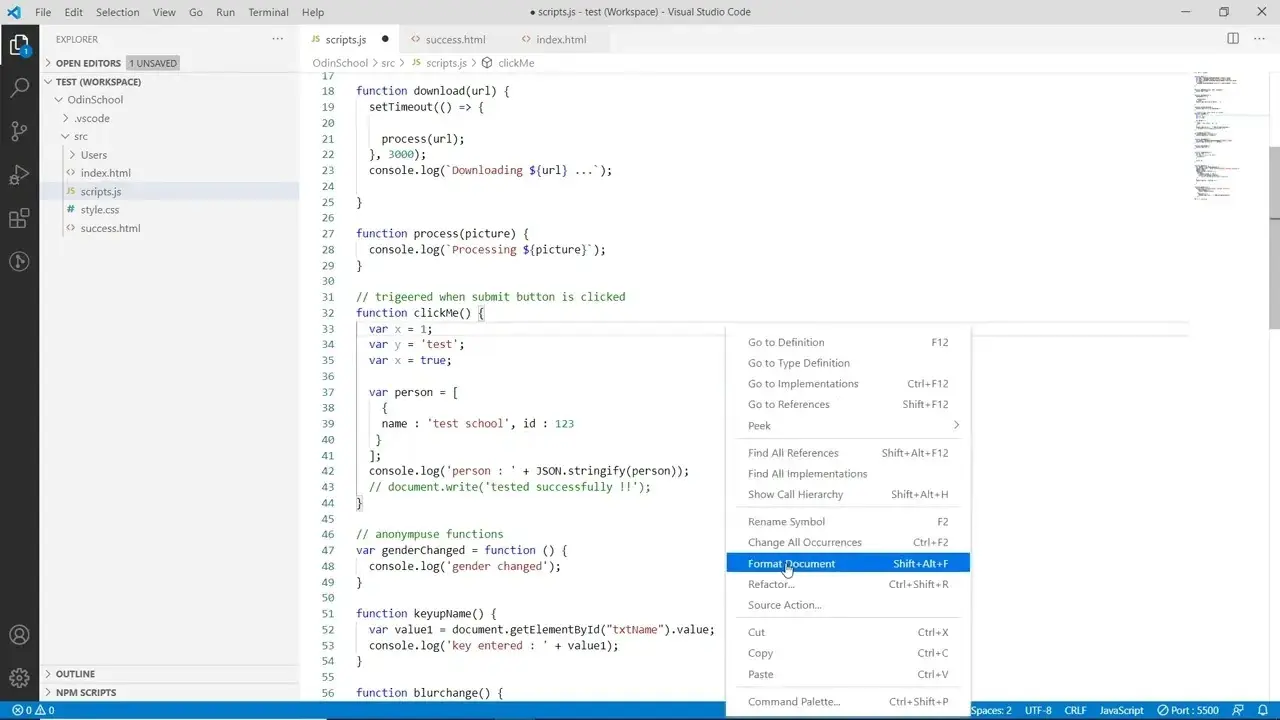

Overview:
Welcome to Module 4 of our web design journey! In this lesson, we'll dive into the world of JavaScript, exploring the power of objects, events, and popup boxes to elevate user interaction and functionality on web pages. By mastering these key concepts, you'll be able to create dynamic and engaging web experiences that captivate your audience.
JavaScript serves as the backbone of modern web development, enabling developers to add interactivity and dynamism to static web pages. In this lesson, we'll delve into JavaScript objects, events, and popup boxes, unlocking the potential to create rich and immersive user experiences.
JavaScript Objects and Functions:
Objects are the building blocks of JavaScript, allowing developers to encapsulate data and functionality into reusable components. Let's explore the fundamentals of objects and functions:
Defining and Using Objects: Objects in JavaScript are collections of key-value pairs, representing real-world entities or concepts. Example:
```javascript
// Defining a person object
var person = {
name: "John Doe",
age: 30,
profession: "Web Developer"
};
```
Constructor Functions and Prototypes: Constructor functions are used to create multiple instances of an object with shared properties and methods. Prototypes enable inheritance and code reuse in JavaScript. Example:
```javascript
// Constructor function for creating person objects
function Person(name, age, profession) {
this.name = name;
this.age = age;
this.profession = profession;
}
// Creating instances of the Person object
var person1 = new Person("John Doe", 30, "Web Developer");
var person2 = new Person("Jane Smith", 25, "Graphic Designer");
```
Object-Oriented Programming Concepts: JavaScript supports key principles of object-oriented programming (OOP), including encapsulation, inheritance, and polymorphism. Example:
```javascript
// Encapsulation: Hiding internal implementation details of an object
function Circle(radius) {
this.radius = radius;
this.getArea = function() {
return Math.PI Add star this.radius add star this.radius;
};
}
// Inheritance: Reusing code and behavior from a parent object
function Square(side) {
this.side = side;
}
Square.prototype = Object.create(Circle.prototype);
Square.prototype.constructor = Square;
// Polymorphism: Objects of different types responding to the same method call
var shapes = [new Circle(5), new Square(4)];
shapes.forEach(function(shape) {
console.log("Area:", shape.getArea());
});
```
Handling Events:
Events are user interactions or system occurrences that trigger JavaScript code execution. Let's explore how to handle events effectively:
Introduction to JavaScript Events: Common events in JavaScript include click, hover, submit, and load. These events enable developers to respond to user actions and modify webpage behavior dynamically.
Event Listeners: Event listeners are used to attach event handlers to HTML elements, allowing developers to execute custom JavaScript code when specific events occur. Example:
```javascript
// Adding a click event listener to a button element
var button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
```
Event Propagation: Event propagation refers to the mechanism by which events are propagated through the DOM tree. JavaScript supports two models of event propagation: bubbling and capturing. Example:
```html
<div id="outer">
<div id="inner">Click me!</div>
</div>
<script>
var outer = document.getElementById("outer");
var inner = document.getElementById("inner");
outer.addEventListener("click", function() {
console.log("Outer div clicked!");
});
inner.addEventListener("click", function(event) {
console.log("Inner div clicked!");
event.stopPropagation(); // Prevents event from bubbling up to the outer div
});
</script>
```
Popup Boxes:
Popup boxes are simple yet powerful mechanisms for displaying alerts, confirmations, and input prompts to users. Let's explore the different types of popup boxes:
- Alert Boxes: Alert boxes display a message to the user, typically used for important notifications or warnings. Example:
```javascript
// Displaying an alert box with a custom message
alert("Hello, world!");
```
- Confirmation Boxes: Confirmation boxes prompt the user to confirm or cancel an action, providing a simple way to gather user input. Example:
```javascript
// Displaying a confirmation box and capturing user input
var result = confirm("Are you sure you want to delete this item?");
if (result) {
// Delete item
} else {
// Cancel action
}
```
- Input Boxes: Input boxes allow users to enter text or numeric input, making them useful for collecting user data or responses. Example:
```javascript
// Displaying an input box and capturing user input
var name = prompt("Please enter your name:");
alert("Hello, " + name + "!");
```
Practical Demonstration:
Let's put theory into practice with a practical demonstration:
- Creating Custom Objects and Functions: Define custom objects and constructor functions to represent real-world entities or concepts in JavaScript.
- Implementing Event Handlers: Attach event listeners to HTML elements and respond to user interactions by executing custom JavaScript code.
- Utilizing Popup Boxes: Display alert, confirmation, and input boxes to enhance user interaction and gather user input effectively.
Conclusion:
In this lesson, we've explored JavaScript objects, events, and popup boxes, unlocking the potential to create dynamic and interactive web experiences. By mastering these key concepts, you'll be able to enhance user interaction and functionality on your web pages, creating engaging and immersive user experiences. Stay tuned for the next lesson, where we'll delve deeper into advanced JavaScript topics and explore techniques for building dynamic web applications. Happy coding!